Recursion
Iteration is a basic computational need in any programming language, yet Haskell doesn't have loops. It doesn't need them, as loops aren't the only way to iterate. Haskell has functions, and functions iterate by recursing. We saw several examples in the last chapter of how to repeat IO actions with impure recursive functions. Since recursion is key part of Haskell, let's examine a few more examples of recursion, this time using pure functions. We'll also consider how to translate a loop-based algorithm into a recursive function.
Loop to Recursion
In computer graphics, image assets tend to have dimensions that are powers of 2. Such images are accessed faster by hardware than images of other dimensions. If an image has dimensions that are not powers of 2, it is padded with extra pixels to reach the next power of 2. For example, this image originally had a resolution of 320×214, but it has been padded out to a 512×256 image:
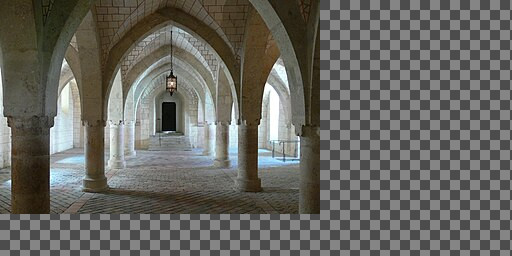
Let's call the next biggest power of 2 of a number its power-of-2 ceiling. The power-of-2 ceiling of 107 is 128. The ceiling of 30 is 32.
In C, we might write the power-of-2 ceiling function with this while loop that doubles a variable until it reaches or just exceeds the target number:
int ceiling2(int target) {
int power2 = 1;
while (power2 < target) {
power2 *= 2;
}
return power2;
}
int ceiling2(int target) { int power2 = 1; while (power2 < target) { power2 *= 2; } return power2; }
The power2
variable is mutated, so we have to make some significant changes to translate it to Haskell. A loop becomes the recursive case of a recursive function. The returned expression becomes the base case. A mutated variable becomes a parameter. Each call to the recursive function passes along the variable's “mutated” value. An equivalent ceiling2
in Haskell might look something like this:
ceiling2 :: Int -> Int -> Int
ceiling2 target power2 =
if power2 >= target then
power2
else
ceiling2 target (power2 * 2)
ceiling2 :: Int -> Int -> Int ceiling2 target power2 = if power2 >= target then power2 else ceiling2 target (power2 * 2)
The C version only required one parameter, but the Haskell version requires two. Adding a parameter makes the function harder to use. On the initial call to the Haskell function, the second parameter must be seeded with 1. Expecting a human to properly seed recursive parameters correctly is a big ask. A safer approach is to make the two-parameter function a hidden helper function and expose a simpler, one-parameter function that calls the helper function with the correct seed. This implementation uses a where
clause to hide the function:
ceiling2 :: Int -> Int
ceiling2 target = helper 1
where
helper power2 =
if power2 >= target then
power2
else
helper (power2 * 2)
ceiling2 :: Int -> Int ceiling2 target = helper 1 where helper power2 = if power2 >= target then power2 else helper (power2 * 2)
This function demonstrates the three steps needed to translate a looping function into a recursive one:
- Rewrite the loop as a conditional statement. The condition chooses between the base case and recursive case.
- Add each mutated variable as a parameter.
- Turn the loop body into the recursive case. Instead of mutating the variables, make a recursive call with the new values as the actual parameters.
- Turn the returned expression into the base case.
- Hide the function inside one that has a simpler interface and seeds the initial values of the parameters.
Recursing on Lists
A common way to apply a computation to a linked list is to break off the singular head element, process it individually, and recurse on the plural tail as needed. This contains
function, for example, iterates through and compares each head element to a target value:
contains :: Eq a => a -> [a] -> Bool
contains target items =
if null items then -- equivalent to items == []
False
else if target == head items then
True
else
contains target (tail items)
contains :: Eq a => a -> [a] -> Bool contains target items = if null items then -- equivalent to items == [] False else if target == head items then True else contains target (tail items)
It stops recursing when it hits the empty list or when it finds the target.
Function contains
processes an existing list and returns a simple scalar value. What if we have an algorithm that builds a new list? A common strategy is to have each recursive call prepend a single element onto the list returned by a recursive call. Prepending is done using the cons operator :
. The base case returns the empty list to which everything else is prepended.
For example, suppose we want to generate a list containing a given number of zeroes. The length of the list n
isn't known statically, so we can't use a list literal like [0, 0, 0]
. Instead, we generate the list dynamically, one zero at a time. A list of zero zeroes is []
. A list of one is 0 : []
. A list of two is 0 : 0 : []
. A list of three is 0 : 0 : 0 : []
.
We need a recursive function that performs this prepending n
times. Like this one:
zeroes :: Int -> [Int]
zeroes n =
if n == 0 then
[]
else
0 : zeroes (n - 1)
zeroes :: Int -> [Int] zeroes n = if n == 0 then [] else 0 : zeroes (n - 1)